- 프로젝트 기획 노션 링크 : https://www.notion.so/4-SA-e1827ff77716456ca46579105da363fe
- 프로젝트 발표 노션 링크 : https://www.notion.so/Grow-Together-9745b27d341a428a90d777bd7db5bfc3
1️⃣ 롤링페이퍼 작성이 한번만 되는 에러
- 원인) 작성시 닉네임과 롤링페이퍼 내용이 requestDto로 설정되어 있는데, 닉네임이 유니크키로 설정되었기 때문에 하나 이상의 롤링페이퍼를 작성할 수 없었음.
- 해결) 닉네임 유니크키 해제 후 정상 작동
기본키와 유니크키 차이점
- 기본키는 즉, 프라이머리 키는 해당 테이블의 식별자 역할을 합니다. 바로 이 제약조건으로 테이블에 하나만 지정할 수 있습니다. 예를 들면, 사람을 식별할 때는 사람의 이름 대신 주민등록번호를 사용합니다. 그 이유는, 바로 유일한 성질을 가지고 있기 떄문입니다. 이렇게 중복성이 없는 유일성을 가진 성질을 의미합니다. 그 중 사용자가 선택한 것을 기본키라고 할 수 있습니다.
- 유니크 키는 유일성을 가지기 위해 설정한 것입니다. 따라서 지정이 되면 중복이 되는 것을 제어하는 역할을 하게 됩니다. 에를 들면 회원 이름을 중복으로 설정하지 않게 하는 것입니다. 중복되는 이름의 경우에는 뒤에 숫자를 붙여서 최초의 한 사람만 기입할 수 있도록 하는 것입니다. 결국, 프라이머리 키는 유니크 키의 성질을 포함하는 것을 알 수 있습니다. 그 중에, 설계자가 기본적으로 선택한 키라고 할 수 있습니다. 유니크키는 하나의 테이블에 각각 컬럼마다 지정이 가능합니다. 그러나 프라이머리키는 오직 하나만 설정할 수 있습니다.
2️⃣ 댓글 좋아요 수만 오르고 좋아요 취소는 되지 않는 에러*
- 원인) 쿼리문에서 findByUserIdAndUsername 이라고 명시했지만, ()안 매개변수값 순서를 거꾸로 입력
- 해결) 올바르게 순서 정렬 후 정상 작동
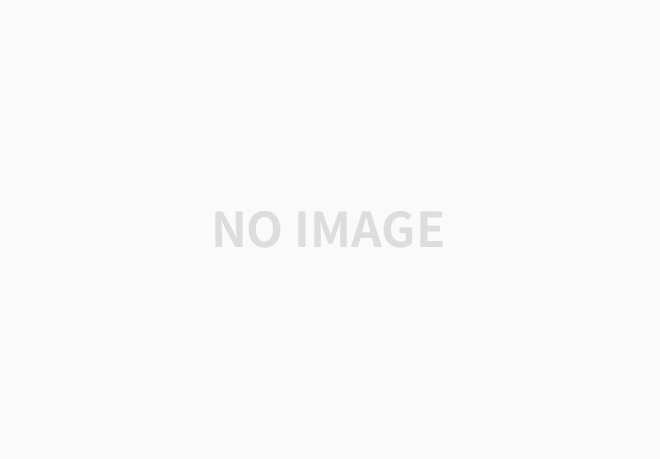
3️⃣ 유효성 검사가 정상적으로 수행되지않지만 에러없이 통과*
- 원인) Build.gradle 파일 내부 dependencies 설정에서 상위버전 valid dependency와 하위버전이 동시에 존재할 경우 dependency간 충돌이 있어 애플리케이션은 에러는 없지만 유효성 검사가 진행되지 않음
- 해결) 사용되지 않거나 중복으로 설정되어진 dependency를 주석처리 또는 삭제하는 것으로 정상적으로 유효성 검사 수행
4️⃣ AWS S3 서버 이미지 업로드 불가
- 문제) S3에 이미지가 로컬에만 저장되고 S3로 convert 되지 않음
- 원인) 추측 - ACL(액세스 제어 목록) 권한설정 문제
- 해결) 추측 - 모든 사람(퍼블릭 액세스) 부분에 객체에 나열 권한을 부여
5️⃣ 예외처리 부분*
- 문제) 모든 에러의 상태코드가 200번으로 반환됨
- 원인) MsgResponseDto로 customException 부분의 메세지부분만 return이 되고 Httpstatus 코드는 반환이 안되고 있었기 때문에 발생함
- 해결) 반환타입을 ResponseEntity로 감싸고 HttpStatus 상태코드도 같이 반환하는 방식으로 변경
6️⃣ CORS 에러*
- 문제) api사용시 cors 에러
- 발생원인) CORS설정을 하지않아 프론트 3000포트에서 백 8080포트의 자원요청 불가
- 해결) 서버에서 CorsConfigurationSource를 통한 CORS 설정으로 해결
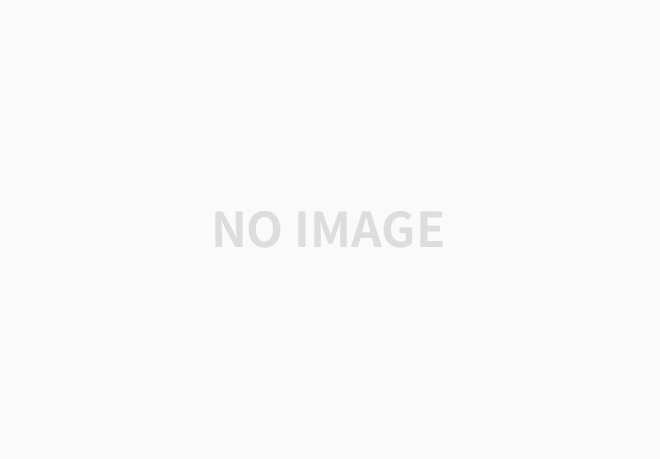
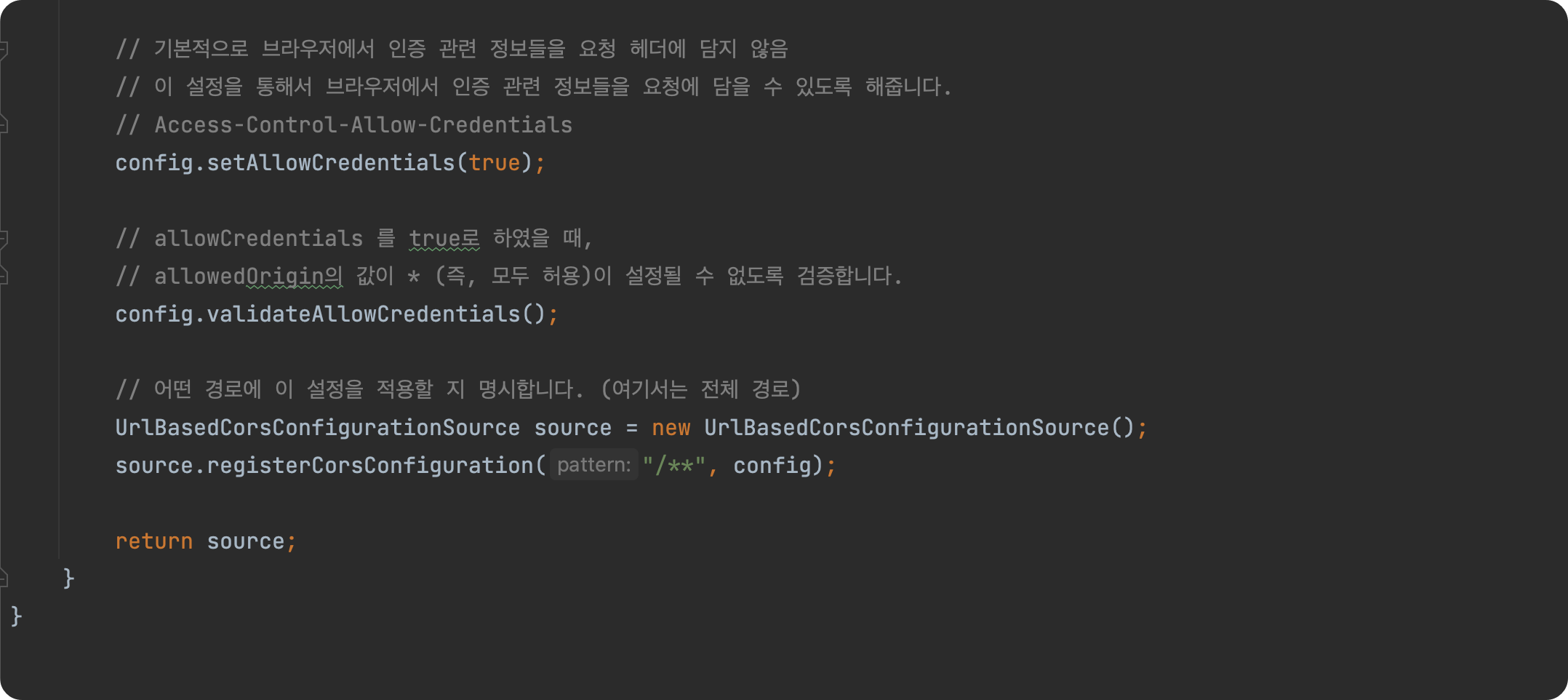
(추가 해결 방안)
- @CrossOrigin 어노테이션 사용 컨트롤러 또는 사용할 메소드에 어노테이션을 추가
- WebMvcConfigurer 설정스프링 프로젝트를 생성하면 @SpringBootApplication 어노테이션이 적용된main함수에서WebMvcConfigurer 을 @Bean으로 등록하여 Cors매핑을 추가
7️⃣ 토큰이 삭제되지 않아 토큰 만료 메세지가 계속 뜨는 에러
- 문제)만료된 토큰을 계속 들고 있어서 만료되었습니다 메세지가 계속 뜸
- 원인)토큰 값이 만료되면 자동적으로 토큰 값을 삭제 해줘 야 되는데 계속 남아있음
- 해결)localStorage.removeItem("token")을 이용하여 status 401 이 뜰 때 if문을 사용하여 제거함
8️⃣ json 에러
- 문제)서버로 데이터를 전송할 때 json문자열로 보내야 하는데 변환되지 않는 에러
- 원인)"Content-Type": "application/json”이 담긴 config를 헤더 값에 넣어주지 못함
- 해결)config 만 해준 곳에 {headers : config} 를 넣어 직접 명시를 해줌으로써 해결
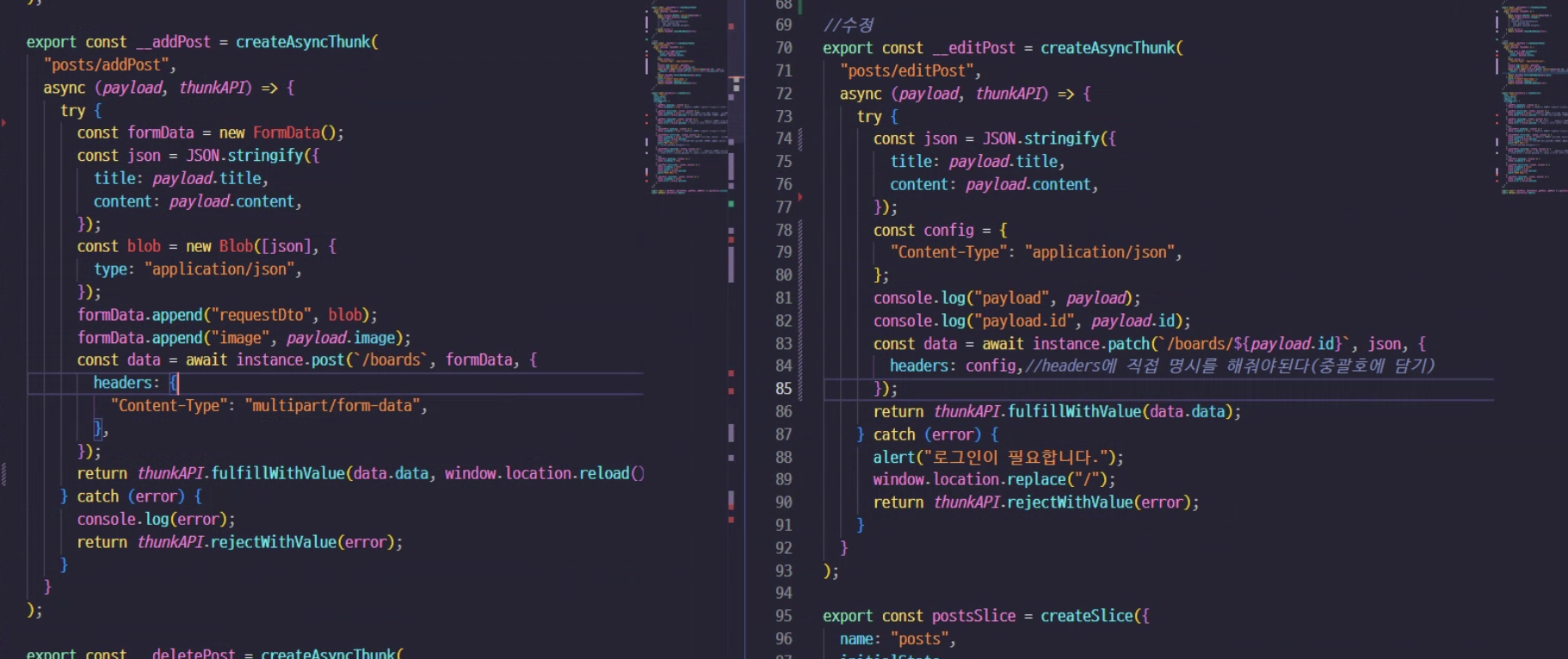
※ 그 외 트러블슈팅 해결 방법 - 프론트엔드 리엑트 코드는 잘 몰라서, 아래와 같이 스프링에 System.out.pringln 을 사용하여 들어가는 값을 확인해 주면서 에러 체크를 하기도 했다.
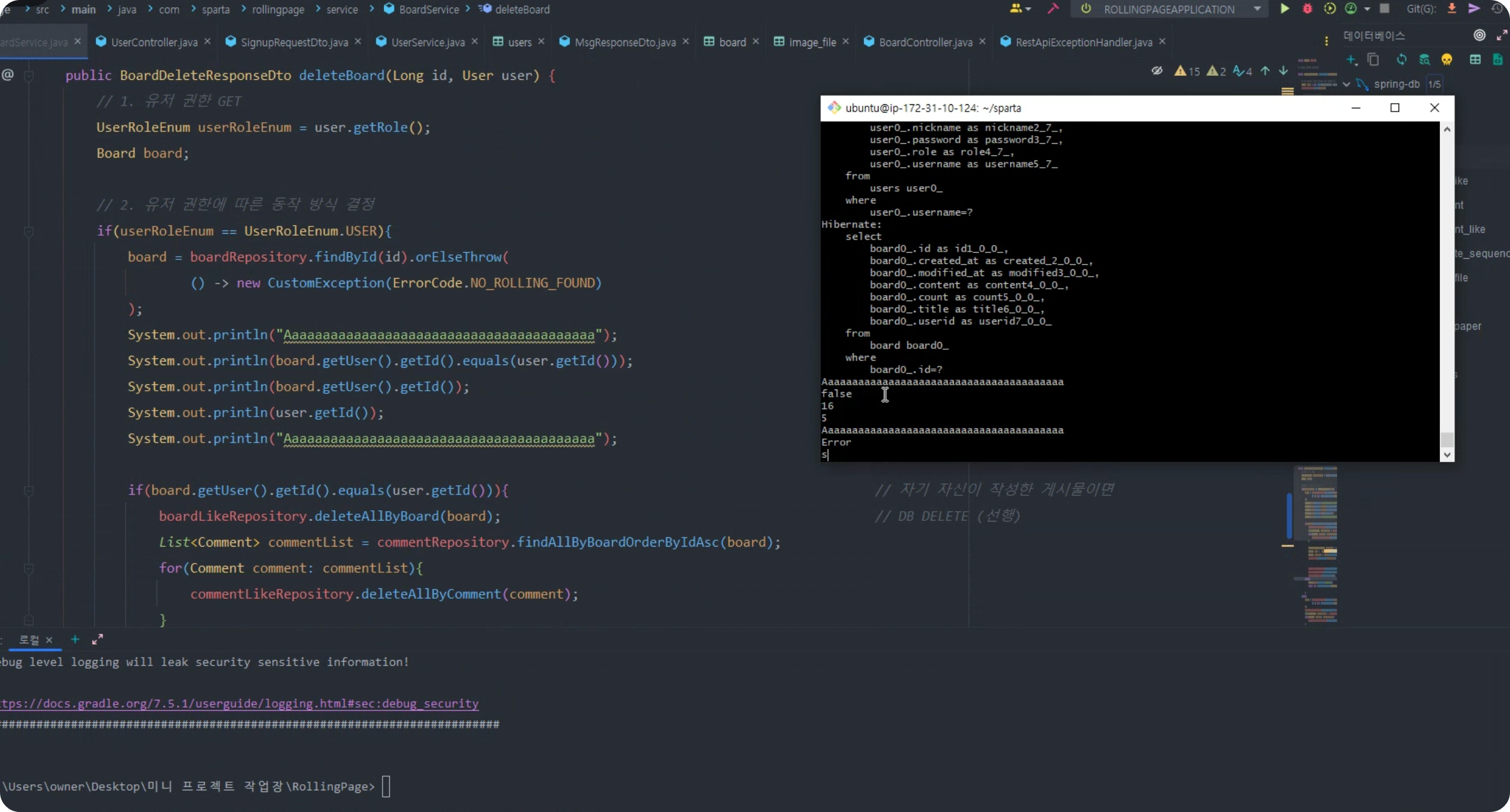
※ 아쉬웠던 부분 / 정리 💡
- 협업은 의사소통이 정말 중요하다. 특히 API 명세를 잘 작성하고 수정이 될 때마다 업데이트를 해줘야 한다.
- 각 파트가 서로의 기능이 완성이 될 때마다 공유를 하며 맞춰가는 과정이 필요했는데 나중에 한꺼번에 합치려다보니 더 힘들었던 것 같다. 완성된 기능끼리는 바로바로 합치는 과정이 필요하다.
'항해99 개발 일지 > [6주차] 미니 프로젝트' 카테고리의 다른 글
[02] S3로 이미지 업로드 / 조회 / 삭제 구현하기 (0) | 2022.12.25 |
---|---|
[01] 프로젝트 기획 (0) | 2022.12.22 |